At production time, you can't debug your application and sometimes errors that appear in the production server don't appear in the development or in preProd server. So if you try to get the error to solve it in production, you need to find a way to help you in getting the error and tracing your application at production time without stopping or using debug mode in application.
Here we will start explaining how to install and configure this tool to work with ASP.NET 2.0, 3.0, 3.5 using C#.
Using
In this article, I'll explain how to use file appender.
Using File appender helps you to print all messages you need in file. The file will be appended to rather than overwritten each time the logging process starts.
Collapse
Our message:
Try to change:
Collapse
Collapse
Collapse
For more information, click here.
Good luck!
Log4net
is a tool to help the programmer output log statements to a variety of output targets in production, PreProd or development server. Here we will start explaining how to install and configure this tool to work with ASP.NET 2.0, 3.0, 3.5 using C#.
Using
log4net
will help you to collect errors in many ways: FileAppender
(collect messages in file)SMTPAppender
(collect messages in email)ADONetApp
(collect messages in database)ender
EventLogAppender
(collect messages in event viewer)
In this article, I'll explain how to use file appender.
Using File appender helps you to print all messages you need in file. The file will be appended to rather than overwritten each time the logging process starts.
Using the Code
Let's start:- Create your website application. Let's name it
MyloggerSite
- Download the DLL file from Apache web site (http://logging.apache.org/log4net/download.html)
After downloading extract the file. - After downloading and creating your application, you need to add
Log4net
DLL reference to your web site, right click in your application and choose add reference...
Then click browse tab.
Point to the folder you just extracted and choose the DLL file in this path:
- Now let's configure our application by adding some tags to web.config file: Inside
<configSections>
tag, add this tag:
Collapse
<section name="log4net" type="log4net.Config.Log4NetConfigurationSectionHandler, log4net"/>
- After
</system.web>
closing tag, we will add a new tag forLog4net
.
Collapse
<log4net> <appender name="FileAppender" type="log4net.Appender.FileAppender"> <param name="File" value="c:\\ MyloggerSite.log"/> <!-- Example using environment variables in params --> <!-- <param name="File" value="${TMP}\\ApplicationKit.log" /> --> <param name="AppendToFile" value="true"/> <layout type="log4net.Layout.PatternLayout"> <param name="ConversionPattern" value="%d [%t] %-2p %c [%x] - %m%n"/> </layout> </appender> <root> <level value="ALL"/> <appender-ref ref="FileAppender"/> </root> </log4net> In<root> <level value="ALL"/>
You can change all values to any of this list:
- ALL
- DEBUG
- INFO
- WARN
- ERROR
- FATAL
- OFF
- Now we need to add Global Application class to our application (Global.asax). Open your Global.asax file and add the following code in the
Application_Start()
method:
Collapse
void Application_Start(object sender, EventArgs e) { log4net.Config.DOMConfigurator.Configure(); }
- Now your
log4net
is ready to use.
log4net
library.
using log4net;
using log4net.Config;
then lets declare the logger var.
ILog logger = log4net.LogManager.GetLogger(typeof(_Default));
//ILog logger = log4net.LogManager.GetLogger("type any thing you want");
But better to use the class file as I did first [typeof(_Default)].
ILog logger = log4net.LogManager.GetLogger(typeof(_Default));
protected void Page_Load(object sender, EventArgs e)
{
logger.Info("Hello Nine Thanks for use Log4Net,This is info message");
logger.Debug("Hello Nine Thanks for use Log4Net,This is Debug message");
logger.Error("Hello Nine Thanks for use Log4Net,This is Error message");
logger.Warn("Hello Nine Thanks for use Log4Net,This is Warn message");
logger.Fatal("Hello Nine Thanks for use Log4Net,This is Fatal message");
}
Open your C: drive and you'll see a file with the name MyloggerSite.log is created and our message has been added to it. 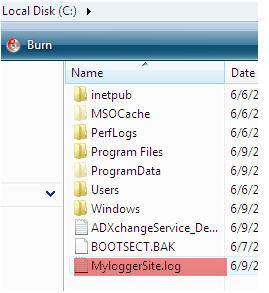


<root>
<level value="ALL"/>
to: 
<root>
<level value="Error"/>
The last thing we need to explain here is the message format we printed: 
<param name="ConversionPattern" value="%d [%t] %-2p %c [%x] - %m%n"/>
<param name="ConversionPattern"
value="%date [%thread] %-5level %logger [%ndc] - %message%newline" />
Compare these two lines respectively. For more information, click here.
Good luck!
Awsum post dude...
ReplyDelete